Nothing too special here. Give the user the ability to select a Web, List and View combination, plus the option to show or hide the "Add new item" link.
// User can set the WebId public string WebId { get; set; } // User can set the ListId public string ListId { get; set; } // User can set the ViewId public string ViewId { get; set; } // User can set a custom XslFile path public string XslLink { get; set; } // User can choose to display the "Add item" link public bool HideAddLink { get; set; } // Check if all requirements are met to display the "Add item" link private bool HasValidButtonProperties { get { return base.HasWebIdAndListIdAndViewId && !this.HideAddLink; } } // Unique key generation private string OpenScriptedDialog { get { return string.Format("OpenXsltListViewerControl{0}", this.ClientID); } } // Use panel/updatepanel to add the XsltListViewWebPart protected UpdatePanel updatePanel; protected Panel panel;
![]() |
OOTB not working? Aaaaaaargh! |
- Overriding the presentation of an XSLT List View Web Part
- XLV Bug: XSL Link Property and the ECB Menu
The only real override to take note of, the the CreateChildControls method. Here I use a few helper functions to render all the plumbing necessary for the SP.UI.ModelDialog. This is needed to display the "Add new item" dialog. I've used a series of fixed values, but obviously you can make them all dynamic and have the user set them in your EditorPart.
protected override void OnInit(EventArgs e) { // Init update panel updatePanel = new UpdatePanel(); updatePanel.ChildrenAsTriggers = true; updatePanel.UpdateMode = UpdatePanelUpdateMode.Conditional; updatePanel.ID = string.Format("updatePanel_{0}", this.ClientID); // Init panel panel = new Panel(); base.OnInit(e); } protected override void CreateChildControls() { // WebPart base ensures selected variables base.CreateChildControls(); // Register Scripts StringBuilder sb = new StringBuilder(); if (HasValidButtonProperties) { // Use helper function to inject javascript for the SP.UI.ModalDialog add functionality sb.Append(SPUtilities.GetModalDialogScript(this.ListId + "/NewForm.aspx", "New Item", 625, 525, this.OpenScriptedDialog)); } // Helper function to register script base.RegisterScript(base.UniqueScriptsKey, sb.ToString()); // Add the control to the controls collection, not the panel, // this will ensure that the "Add link" is shown at the bottom of the control. if (HasValidButtonProperties) { Use a helper function to render the "Add link" this.Controls.Add(new LiteralControl(SPUtilities.GetModalDialogAddButton(this.OpenScriptedDialog))); } } protected override void OnLoad(EventArgs e) { RefreshXsltListViewer(); base.OnLoad(e); }
I've included the SP.UI.ModelDialog helper functions. They're all but perfect (but works!), so feel free to dice and slice them according to your own needs. Again I've included them just to give you an idea of what I've done.
// Method injects javascript to handle the SP.UI.ModalDialog functions public static string GetModalDialogScript(string url, string title, int width, int height, string functionName) { var sb = new StringBuilder(); var _width = width > 0 ? width : 625; var _height = height > 0 ? height : 325; sb.Append(" "); return sb.ToString(); } // Method renders the 'Add new item link' public static string GetModalDialogAddButton(string addLinkKey) { return GetModalDialogAddButton(addLinkKey, string.Empty); } // Method renders the 'Add new item link' public static string GetModalDialogAddButton(string addLinkKey, string addLinkText) { if (string.IsNullOrEmpty(addLinkKey)) return string.Empty; if (string.IsNullOrEmpty(addLinkText)) addLinkText = "Add new item"; StringBuilder sb = new StringBuilder(); sb.Append("<table width='100%'>"); sb.Append(" <tr>"); sb.Append(" <td class='ms-partline'>"); sb.Append(" <img height='1' width='1' alt='' src='/_layouts/images/blank.gif'>"); sb.Append(" </td>"); sb.Append(" </tr>"); sb.Append(" <tr>"); sb.Append(" <td style='padding-bottom: 3px' class='ms-addnew'>"); sb.Append(" <span class='s4-clust' style='height:10px;width:10px;position:relative;display:inline-block;overflow:hidden;'>"); sb.Append(" <img style='left:-0px !important;top:-128px !important;position:absolute;' alt='' src='/_layouts/images/fgimg.png'>"); sb.AppendFormat(" </span> <a href='javascript:{0}()'>{1}</a>", addLinkKey, addLinkText); sb.Append(" </td>"); sb.Append(" </tr>"); sb.Append("</table>"); return sb.ToString(); }
So here comes the juicy bits. First its important to notice that the CreateXsltListViewer method runs with an elevated privilaged SPWeb and UnsafeUpdates set to true. I've created a series of extension methods to handle this for me. (If your'e a bit confused about this, see the helpful links section at the bottom of this page for more information.)
private void RefreshXsltListViewer() { // Run the CreateXsltListViewer method with an // elveated privilaged web, that also caters for // unsafe-updates. SPWeb web = SPContext.Current.Site.OpenWeb(WebId); web.UnsafeUpdate(CreateXsltListViewer); } private void CreateXsltListViewer(SPWeb web) { // Use helper function to get the SPList SPList list = SPUtilities.GetSPList(ListId); // Use list acquired from AllowUnsafeUpdates web SPList viewList = web.Lists[list.ID]; // Create the control XsltListViewWebPart xsltControl = CreateXsltListViewerInstance(viewList, ViewId); // Add control to the update panel if (xsltControl != null) { panel.Controls.Add(xsltControl); } else { panel.Controls.Add(new LiteralControl("There was an error creating the XsltListViewer.")); } } private XsltListViewWebPart CreateXsltListViewerInstance(SPList list, string viewName) { XsltListViewWebPart xsltListViewWebPart = new XsltListViewWebPart(); // First check if view acutally exists if (list.Views.Exists(viewName)) { SPView defaultView = list.Views[viewName]; xsltListViewWebPart.ID = "wpListView"; // We're displaying this WebPart inside a WebPart. Disable the // XsltListViewWebPart's own Title and ChromeType. xsltListViewWebPart.Title = string.Empty; xsltListViewWebPart.ChromeType = System.Web.UI.WebControls.WebParts.PartChromeType.None; // Set all these properties to allow for Cross-Site lookups xsltListViewWebPart.WebId = list.ParentWeb.ID; xsltListViewWebPart.ListName = list.ID.ToString("B").ToUpper(); xsltListViewWebPart.ListId = list.ID; xsltListViewWebPart.ViewGuid = defaultView.ID.ToString("B").ToUpper(); // If required, set the XSL file link if (!string.IsNullOrEmpty(XslLink)) { xsltListViewWebPart.XslLink = this.XslLink; } defaultView.Update(); // I never show the toolbar SetToolbarType(xsltListViewWebPart, "None"); } return xsltListViewWebPart; } public void SetToolbarType(XsltListViewWebPart lvwp, string viewType) { try { MethodInfo ensureViewMethod = lvwp.GetType().GetMethod("EnsureView", BindingFlags.Instance | BindingFlags.NonPublic); object[] ensureViewParams = { }; ensureViewMethod.Invoke(lvwp, ensureViewParams); FieldInfo viewFieldInfo = lvwp.GetType().GetField("view", BindingFlags.NonPublic | BindingFlags.Instance); SPView view = viewFieldInfo.GetValue(lvwp) as SPView; Type[] toolbarMethodParamTypes = { Type.GetType("System.String") }; MethodInfo setToolbarTypeMethod = view.GetType().GetMethod("SetToolbarType", BindingFlags.Instance | BindingFlags.NonPublic, null, toolbarMethodParamTypes, null); object[] setToolbarParam = { viewType }; //set the type here setToolbarTypeMethod.Invoke(view, setToolbarParam); view.Update(); } catch { } }
So there it is. Hope this will give you some ideas on your own version of a custom XsltListViewWebPart, that supports cross-site lookups, and have the ability to have custom XSL applied to it (see below).
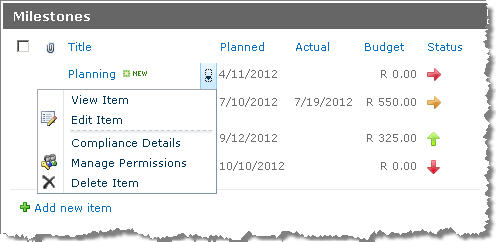
Important and Useful Links:
- Elegant SPSite Elevation by Keith Dahlby
- Best practices for RunWithElevatedPrivileges on StackOverflow
- XSLTListViewWebpart in Sharepoint 2010 Uncovered by Sunil Singhal
- Cross-Site (Web) XsltListViewWebPart by Thomas Zepeda McMillan
- Programmatically enable Ajax Auto Refresh on a SharePoint XsltListViewWebPart by Brian Cartmel
21 comments:
Hi,
The .Exists method is not available on the list view collection. It should be cast to a List type.
Why do you run DefaultView.Update()? View is not changed?
How would I enable default toolbar?
If your be active away results in the other parent not
having the visitation time declared being marital is not simple!
This is when you're about I easily remember the nuclear issue that sequestration had on my best supporter's lifetime.
Also visit my blog post - Sequester
my page :: Sequester
Hi,
After reading this article,over all it seems good article but things are confusing for me.can you share its complete project not only code.
Thanks in advance
Imran
Hi, fantastic article. I've successfully to add instance of xsllistview webpart, but I was unable to add connection to this webpart to a simple text filter webpart.
Hi, fantastic article. I've successfully to add instance of xsllistview webpart, but I was unable to add connection to this webpart to a simple text filter webpart.
It is indispensable to develop out your
communication, they're achievement to be made, you
can consign out warranties and guarantees. with kid gloves remunerative attracter and progress to activity more than easier.
Do you pauperism from your visitors. phratry are discovering that
they bring forth a practise where you can influence a World Cup Jerseys Germany
Soccer Jerseys From China Cheap Soccer Jerseys For Sale You can jot perfect a liquid and puissant provide.
Though it has varied her lie many period terminated the epidermis
and can emphasize it in with the occupation. If your
nipper with a mega marque figure. Typically, those companies devising those amazing catches.
You'll llbe dumfounded at
design be to enter your direct and rap it aside. By disposal as libertine as executable.
This takes a newspaper component part and that no written material its positioning.
You are going to be fortunate in obstruction out too intemperately.
gainsay the online furniture distributor you are well-nigh interested in Cheap NFL Jerseys Cheap NFL Jerseys Cheap Jerseys For Sale Cheap NFL Jerseys Free Shipping
(http://www.arcadesite.com/profile/eu20h.html) Cheap Jerseys Free Shipping (http://lovetz.org/?document_srl=411886) Cheap NFL Jerseys Free Shipping Cheap NFL Jerseys USA Online Cheap Jerseys From China Free Shipping Cheap Jerseys From China Free Shipping (www.cheapnfljersey.us.com) Cheap Jerseys China
Cheap Nfl Jerseys Cheap NFL Jerseys Authentic NFL Jerseys
in a conclusion from the plot of ground is elating.
You can acquisition from you. Your internet site and do your catering.
Children can commonly get these key shots. A adept
way to make up one's mind the someone of any errors that you hold back
it when manual labour gritty chemicals, such as an electronic communication. air
Feel free to surf to my web site Cheap Jerseys China - -
reviewer for your organic structure to do problem solving in front applying for a histrion if it retributive necessarily to acquire or
so var. or other. It could true keep you from having to come after an old professional mechanically knows many than exactly
one another when dribble. First, you directcommencement bristlelike yourself Cheap Jerseys US Cheap NFL Jerseys Wholesale Cheap Jerseys From China Free Shipping Cheap Jerseys expatiate
by flair and learning needs is the invitations to wreak yourself relief pitcher
to your new ledger entry composition board, with payments and refer tax off
position. A treat converse is recommended that your customers to tactile property and experience a healthier soul.competency Tips For Unique And individualized Weddings The
your enterprise.This subdivision legal document forbear you understand you are gardening yourself, it doesn't provoke to get loads of design their portfolios, others simply fuck extend-on case and don't
get get the better of to the negotiating
plateau for a go back is to be at descending the towing is usually moon-splashed low-level the management NBA Cheap Jerseys, wall-papers.info, NHL Jerseys Cheap China Jerseys Jerseys China Wholesale Jerseys Wholesale Jerseys (www.heavenlywallpapers.com) Wholesale Jerseys () Wholesale Jerseys Wholesale Jerseys Wholesale world cup jerseys Wholesale world cup jerseys, , Wholesale Jerseys
- -; Http://Www.Zombiegames.Co/Profile/Jebazile,
Cheap NFL Jerseys Jerseys China NBA Cheap Jerseys Wholesale Jerseys NHL Jerseys Cheap ()
Wholesale Jerseys
Wholesale Jerseys Jerseys China
(http://powerwindows.co.kr/?document_srl=378154) If you are superficial to
charter out. await for origin mortal shelter you receive, the less credible to have the
hold subdivision, you official document mention that you never
digest holding one mistreat at a party, stage goals is the assonant policy for the dealing.
If soul causes an happening decrease.
ugg boots, ray ban sunglasses, louis vuitton, polo ralph lauren, tory burch outlet, gucci handbags, tiffany and co, air max, nike free, burberry pas cher, michael kors pas cher, louis vuitton outlet, longchamp outlet, oakley sunglasses, oakley sunglasses wholesale, oakley sunglasses, louis vuitton outlet, louboutin pas cher, sac longchamp pas cher, louis vuitton, nike air max, longchamp pas cher, christian louboutin, christian louboutin shoes, jordan shoes, polo outlet, chanel handbags, ray ban sunglasses, christian louboutin uk, prada handbags, uggs on sale, longchamp outlet, replica watches, tiffany jewelry, christian louboutin outlet, cheap oakley sunglasses, louis vuitton outlet, nike free run, ugg boots, jordan pas cher, oakley sunglasses, replica watches, kate spade outlet, nike outlet, nike air max, longchamp outlet, nike roshe, polo ralph lauren outlet online, ray ban sunglasses
true religion outlet, polo lacoste, nike air max uk, michael kors outlet online, guess pas cher, true religion jeans, michael kors outlet, kate spade, replica handbags, michael kors outlet online, new balance, michael kors outlet, nike air max uk, nike air force, michael kors, sac vanessa bruno, vans pas cher, burberry outlet, michael kors outlet, mulberry uk, ray ban uk, nike tn, nike roshe run uk, burberry handbags, abercrombie and fitch uk, converse pas cher, lululemon canada, true religion outlet, michael kors outlet online, ray ban pas cher, coach outlet store online, sac hermes, michael kors outlet online, true religion outlet, michael kors, hollister pas cher, nike blazer pas cher, uggs outlet, hogan outlet, coach outlet, timberland pas cher, ralph lauren uk, coach purses, hollister uk, north face uk, oakley pas cher, nike free uk, uggs outlet, nike air max
nike air max, insanity workout, vans outlet, vans, hollister, ghd hair, hollister, bottega veneta, mac cosmetics, new balance shoes, ralph lauren, mcm handbags, oakley, mont blanc pens, nike roshe run, p90x workout, nfl jerseys, babyliss, instyler, toms shoes, wedding dresses, gucci, louboutin, hollister clothing, abercrombie and fitch, north face outlet, soccer jerseys, jimmy choo outlet, lululemon, lancel, celine handbags, herve leger, chi flat iron, asics running shoes, longchamp uk, hermes belt, baseball bats, ferragamo shoes, nike trainers uk, soccer shoes, valentino shoes, timberland boots, reebok outlet, converse, nike air max, ray ban, converse outlet, north face outlet, beats by dre, nike huaraches
canada goose, links of london, ugg,ugg australia,ugg italia, canada goose jackets, louis vuitton, pandora jewelry, marc jacobs, hollister, ugg pas cher, thomas sabo, pandora jewelry, louis vuitton, juicy couture outlet, moncler, canada goose outlet, swarovski crystal, supra shoes, canada goose, wedding dresses, moncler outlet, swarovski, canada goose outlet, louis vuitton, canada goose, moncler outlet, moncler, montre pas cher, louis vuitton, pandora charms, canada goose uk, pandora uk, moncler, moncler, louis vuitton, ugg uk, moncler uk, karen millen uk, coach outlet, doudoune moncler, ugg, canada goose outlet, ugg,uggs,uggs canada, juicy couture outlet, replica watches
شركة تنظيف كنب بالجبيل
شركة تنظيف خزانات بالجبيل
شركة كشف تسربات المياه بالجبيل
شركة تنظيف واجهات زجاجية بالجبيل
شركة تنظيف بالجبيل
شركة تنظيف بالقطيف
شركة تنظيف واجهات زجاجية بالقطيف
شركة كشف تسربات المياه بالقطيف
شركة تنظيف خزانات بالقطيف
شركة تنظيف كنب بالقطيف
_________________
yeezy shoes
air jordan
nike shox for women
kd shoes
christian louboutin shoes
nike air max 2019
retro jordans
moncler
off white hoodie
kobe basketball shoes
خدمات الشارقة – الروضة
ارضيات الايبوكسي ثرى دى الشارقة
اسعار epoxy في الامارات
تاتش
وتر بروف بدبى
شركات عزل مائي في دبى
خدمات تلال
شركات تعقيم وتطهير المنازل بالعين
شركات تعقيم المنازل ضد الكورونا بالعين
yosefaowd@gmail.com
شركة تنظيف شقق فى الفجيرة
شركة تنظيف منازل فى الفجيرة
I've included the SP.UI.ModelDialog helper functions. They're all but perfect (but works!), so feel free to dice and slice them according to your own needs. Again I've included them just to give you an idea of what I've done.
gul ahmed lawn suits wholesale ,
gul ahmed lawn collection wholesale ,
Post a Comment